Adapter Design Pattern - Illustration and Code
Let's learn about Adapters - a structural design pattern - with an intuitive diagram and matching C# code sampleThe adapter pattern is a structural design pattern that is often used when integrating new code with a legacy library where you wish to avoid updating the old code base, or for example, converting an external XML feed to JSON.
Why C#
For the design patterns series I'll be using C# as I find it an elegant language that makes understanding traditional OOP concepts easier than a language such as Python, which I prefer for algorithmic concepts.
Adapter pattern illustrated
The following illustration is a contrived example of attempting to insert a square into a legacy sorter that can only handle circles, and how the adapter pattern could be used to overcome this.
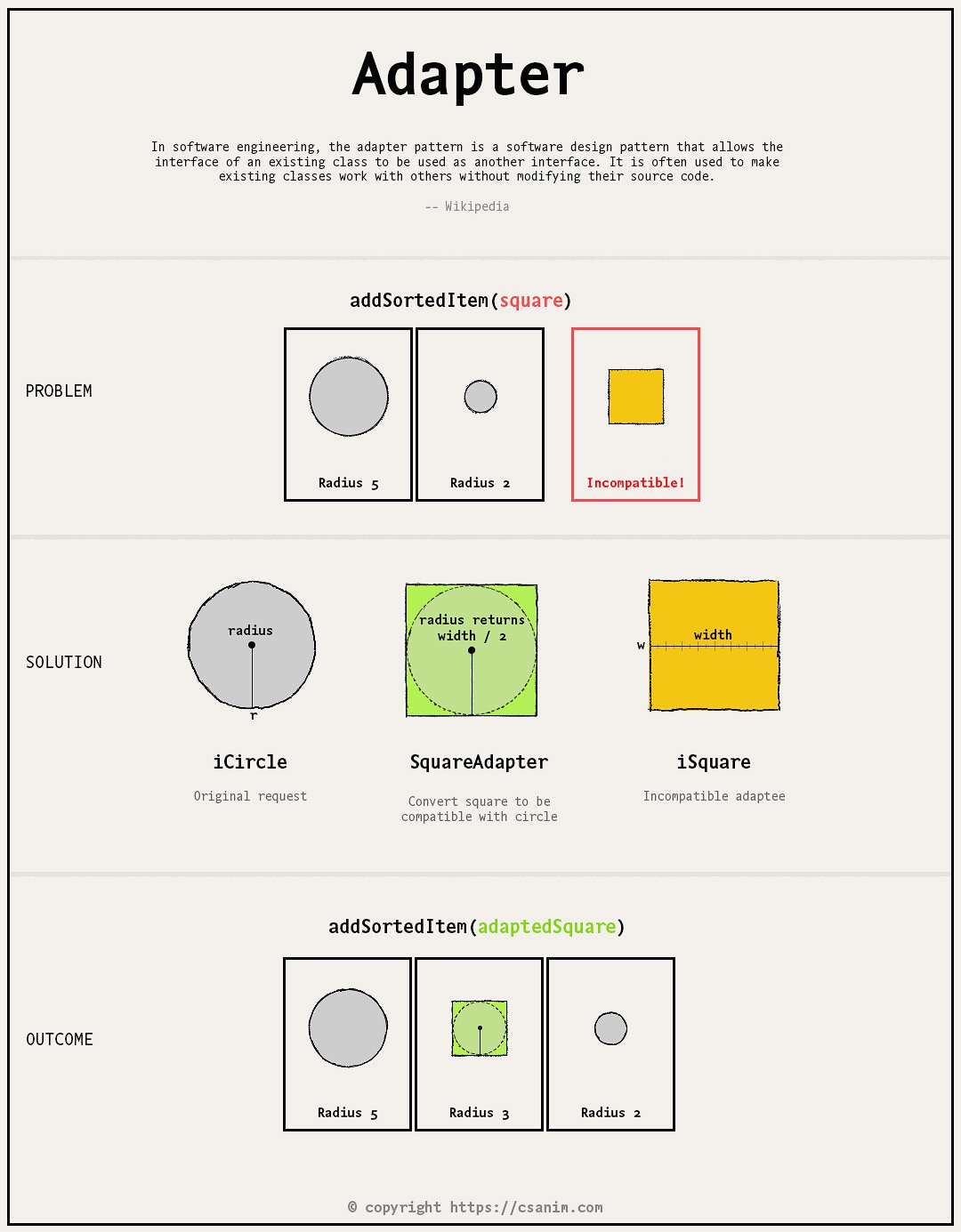
Code example
Let's look at example code for adapting our Square
to be compatible with a LegacySorter
that can only sort circles.
The adaptee
First, we create our adaptee (Square
) that is incompatible with the iCircle
interface:
Adapter
Now we need to create our adapter which implements the original iCircle interface and overrides the iCircle's Radius property with the square's width / 2
value:
Using our new adapter
And finally, we can use our new SquareAdapter
to seamlessly add our square to the legacy sorter:
The end - plus full project code
The adapter pattern is one of the easier patterns to implement and understand, however (as with all design patterns), it can be abused so make sure there is a valid reason to build an adapter rather than simply refactoring a problematic interface.
I've uploaded a full runnable C# code sample to Github here.